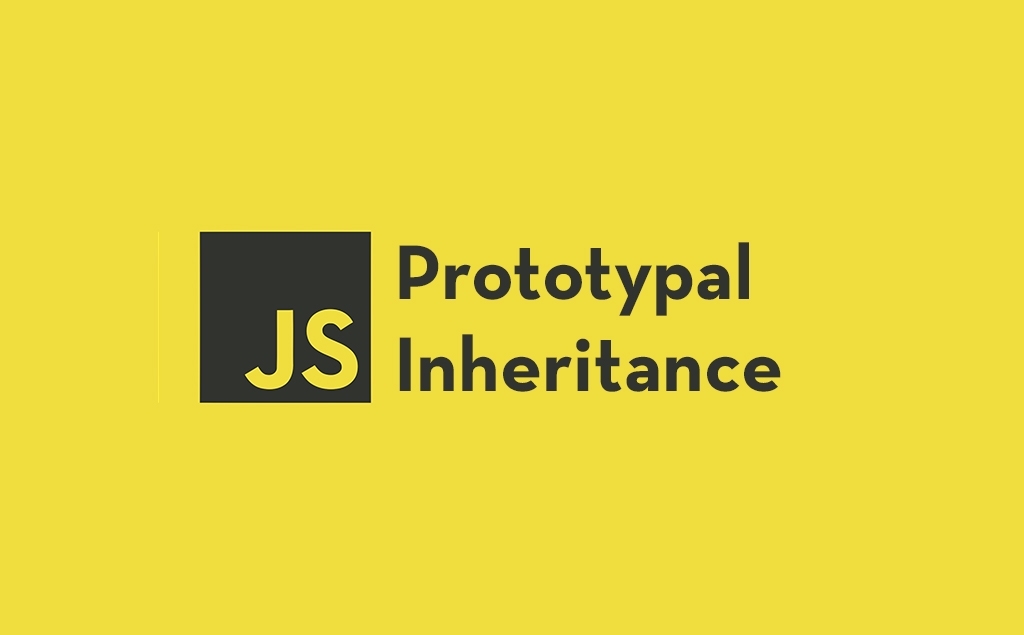
There is a strange syntax called Object.create()
that came out when ES5 was released.
If I want to create an object using inheritance, there is no easier syntax than this.
However, awareness is low due to class
syntax.
Using Object.create()
Object.create(parent object);
If you use it like this, one object data type is left in this place.
And the parent object
written in parentheses becomes the gene (prototype)
.
Let’s take an example.
var parent = { name: "Kim", age: 50 };
var child = Object.create(parent);
console.log(child.age); //50
That’s how you write it.
Then, the child object
will have its parent as prototype
.
If you do child.name
, 'Kim'
will be output, and if you child.age
, 50
will be output.
Did the child successfully inherit parent properties?
This is the end of creating the inheritance function. It’s very simple and easy.
So what if the child wants to change age
?
My son is not 50 years old, but he keeps saying that his age is 50.
Let’s turn this into a 20-year-old.
var parent = { name: "Kim", age: 50 };
var child = Object.create(parent);
child.age = 20;
console.log(child.age); //20
I just gave the value of age: 20
to object
, which is a child.
So now it prints ‘20’ whenever I do it.
Q. Why isn’t the age
of 50
inherited from the parent printed out?
-
How would you respond if the interviewer asked you this Because I learned that there is an order in which to ask when getting certain data out of the JavaScript object data type. If you say, take out
child.age
-
If an
object
called a child directly has anage
, that is displayed. -
If not, check the child’s parent
prototype
and print theage
if it is there. -
If it is not there, search the parent’s parent
prototype
. Printage
in this order.
So now the child comes out with
20
. -
Easy to make even for grandkids.
So it is said that children’s children can easily make it.
Let’s create a grandson that inherits all the attributes of the parent and all the properties of the child.
var parent = { name: "Kim", age: 50 };
var child = Object.create(parent);
child.age = 20;
var grandson = Object.create(child);
console.log(grandson.age);
made grandchildren.
This is a child who inherits all the attributes of both the child and the parents.
So what do we get when we run grandson.age
?
‘20’ is printed.
-
If you still wonder why 20 is coming out
-
Check if the grandchild has
age
, and -
If not, check if it exists in parent
prototype
-
If it’s not there either, check if it’s in the parent’s parent
prototype
and… This is because it checks one by one and outputs the closestage
.
-
Anyway, this is how to inherit inheritance. Very simple, right?
Adding values is as easy as handling object
.
Adding a function is easy because you just put it in an equal sign.
However, these days, developers apparently use class
and extends
syntax to create inheritance of inheritance.
People familiar with programming languages like Java will find it familiar.
There is a lot to memorize, so we may feel a bit reluctant.
▲ In particular, you can sometimes see the extends
syntax when looking at the code written with the old React syntax.