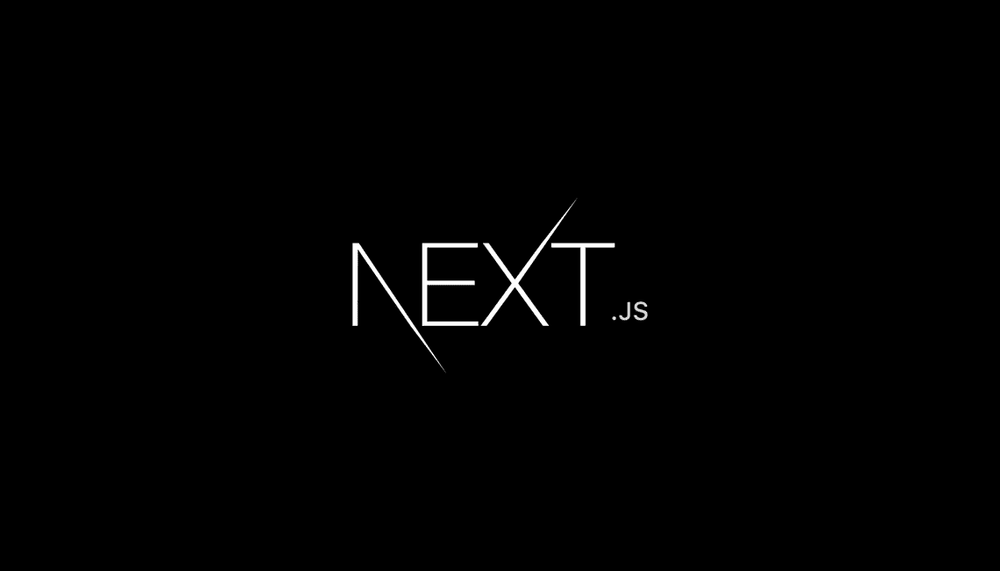
Connecting to MongoDB in a Next.js environment
Next.js version
"next": "13.2.4”
Connecting to MongoDB in a Next.js environment
1. Open a terminal and install the mongodb library.
npm install mongodb
Open a new terminal in your work folder and type this to install it.
This is a library to help you connect to MongoDB
and write to and from it.
const client = await MongoClient.connect('DBconnectURL~~', { useNewUrlParser: true })
const db = client.db("forum")
db.collection().something~
Once installed, you can now write code like this to get data into and out of the DB.
The above 2 lines only need to be executed once, not every time for each input/output.
So let’s wrap the above 2 lines of code in a function and reuse it.
Create a function when you want to shorten a long piece of code into a short word.
2. Create a JS file somewhere and use the
import { MongoClient } from "mongodb";
const url = "DBconnectURL~~";
const options = { useNewUrlParser: true };
let connectDB;
if (process.env.NODE_ENV === "development") {
if (!global._mongo) {
global._mongo = new MongoClient(url, options).connect();
}
connectDB = global._mongo;
} else {
connectDB = new MongoClient(url, options).connect();
}
export { connectDB };
Let’s write it down like this.
-
I created a util folder in my project folder and created database.js in it, and wrote down: - The DB connection URL is the one I just copied from mongodb.com.
-
Description of this code You can create a
connectDB
variable to store the results ofMongoClient.connect
andexport
them to use whenever you need them- but in the case of
Nextjs
development, the javascript files are re-executed every time you save the file.
Multiple
MongoClient.connect
s can be running at the same time, which can make DB input/output very slow.If you want to prevent this, you can use an if statement to say “If you are in development, please keep it in a global variable store called global”.
I don’t want to use global in production, so I added an else statement.
In production, I don’t have a lot of duplicate executions, so it works just fine.
Now whenever you use
await connectDB
, you’ll haveMongoClient(url, options).connect()
in its place, so you can use it however you like. - but in the case of
Then apply and use it as follows
import { connectDB } from "../../util/database";
import DetailBtn from "components/DetailBtn";
import ListItem from "./ListItem";
export default async function List() {
let client = await connectDB;
const db = client.db("forum");
let result = await db.collection("post").find().toArray();
const results = JSON.parse(JSON.stringify(result));
return (
<div className="list-bg">
<ListItem result={results} />
<div>
<div>List</div>
<DetailBtn />
</div>
</div>
);
}
-
Description of this code The
JSON.parse()
function converts a JSON-formatted string to a JavaScript object.The
JSON.stringify()
function converts a JavaScript object to a JSON-formatted string.So,
JSON.stringify(result)
converts theresult
object to a JSON-formatted string, which is then converted back to a JavaScript object using theJSON.parse()
function.This converts all property values within the object to strings, and potentially missing property values in the object’s prototype chain.
However, it is most commonly used to deep copy an object by duplicating it, parsing it back to a JSON-formatted string, and returning the resulting object.
This ensures that the object is passed safely and that the data passed between the server and client is formatted uniformly.