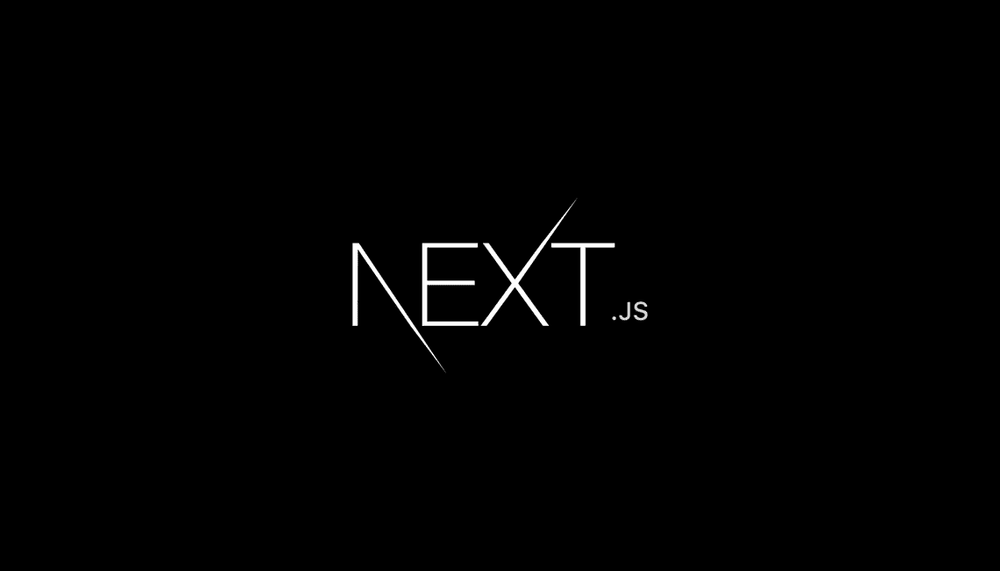
Developing Next.JS Server Features
Next.js version
"next": "13.2.4”
Let’s create a feature that allows users to create posts.
-
Create a page where you can write posts
-
You can send your posts to the DB and store them there.
But there’s a problem. What if a user puts in some weird data?
You need a server
Saving user input directly to the DB can cause big problems.
This is because users might put weird data into the DB.
In fact, the opposite is also dangerous.
If you allow users to print data directly from the DB, what if they print all the sensitive information?
So when you need to access the DB, you write a program that can safely do it for you.
and the user only needs to ask the program to do the DB input/output.
How to create a server
For example, if you wanted to create a feature that saves posts to the DB, you could code something like
“When someone sends you a post title and content, save it to the DB” and you’re done.
However, in server development, there may be many similar functions.
To avoid confusion, each function is labeled with a URL and method name.
- The URL can be anything you want to make it, /anyway /something else
- The method can be any of the four
GET
POST
PUT
DELETE
.
Functions that usually deal with data output include GET
,
POST for input
PUT
for modification
and DELETE
for deletion.
for deletion.
So your server code would be “When someone sends you a post title and content, save it to the DB”.
You write a URL and a method, and you’re done.
The functionality you create on the server is sometimes referred to as an API
.
To create a server function in Next.js
- Create an api folder inside the
pages
folder and create a JS file there with a random name.
For example, I created pages/api/test.js
.
The files and folders you create inside the pages/api/
folder will automatically become the URL of your server function.
So now when a user makes a GET
/POST
/PUT
/DELETE
request to the URL /api/test, the code in test.js
will be executed.
export default function handler(req, res) {
console.log(123);
}
- To be precise, if you put a function in the
test.js
file
The code in that function will be executed when a GET, POST, PUT, or DELETE request is made to /api/test
.
- When a server receives a request, it’s good to respond.
When the server finishes executing your code, make sure to respond.
Otherwise, the requestor could be stuck in an infinite waiting state.
export default function handler(req, res) {
res.status(200).json("Processed");
}
- If the server function ran successfully, put
200
instatus()
.
If the server function failed, put 500
in status()
.
If the user made a bad request and it failed due to user error, you can put 400
.
- If you want to send data to the requestor as well, you can put it inside the
res.json()
.
Send any object
, array
, character
, or number
To make a
POST
request to the server
The <form>
tag makes it easy for users to send POST
and GET
requests to the server.
To do this, let’s create a file called app/write/page.js
.
app / write / page.js;
export default function Write() {
return (
<div>
<h4>Composing posts</h4>
<form action="/api/test" method="POST">
<button type="submit">Button</button>
</form>
</div>
);
}
In the action
field, you can enter a URL, and in the method
field, you can enter either GET
or POST
(but not PUT
or DELETE
).
The request will then be sent to the server when you hit send.
import { connectDB } from "util/database";
const handler = async (req, res) => {
if (req.method == "POST") {
const { title, content } = req.body;
if (title == "" || content == "") {
return res.status(200).redirect(302, "/write");
}
const db = (await connectDB).db("forum");
const result = await db.collection("post").insertOne({
title,
content,
});
console.log(
"The user has entered information ->" +
"title" +
title +
" " +
"content" +
content
);
return res.status(200).redirect(302, "/list");
} else {
return res.status(400).json("This is an incorrect approach.");
}
/**
* If the DB is down or the internet is disconnected or something like that,
errors may occur in the DB. If you want to check for such cases, let's use try, catch.
The try, catch syntax is try { } If the code inside the catch fails,
the code inside the catch { } will be executed instead.
*/
};
export default handler;
(Note1) If you want to execute different functions for each type of method on the server, you can separate them with an if statement.
(Note2) Since the code is executed on the server side, it doesn’t matter if you write DB input/output code here.