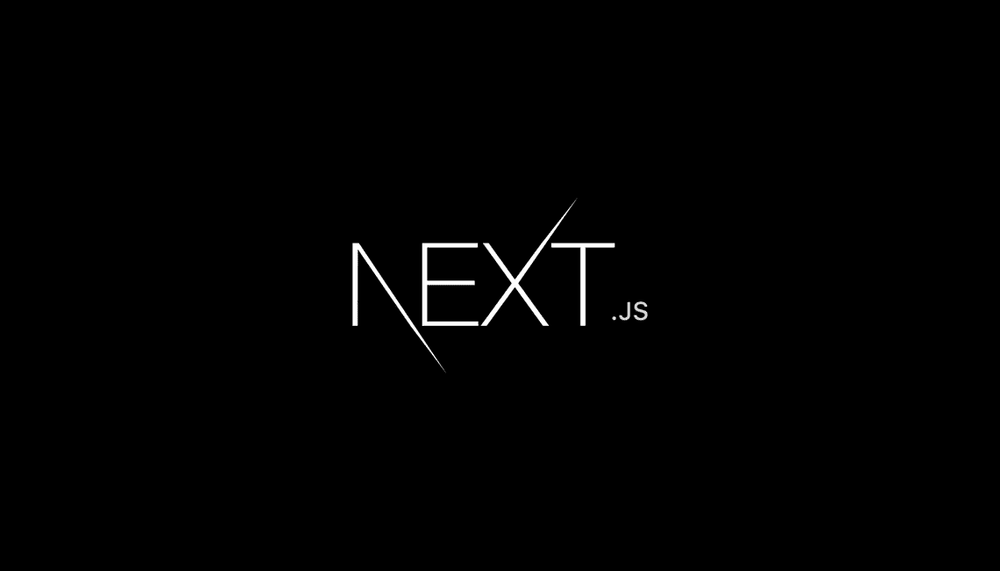
Social login with Auth.js
Next.js version
"next": "13.2.4”
NextAuth Library settings
npm install next-auth
Social login with Github
Create a Github app
Github.com Once logged in, tap Settings -> Developer settings -> Create new OAuth app in the top right corner.
Enter the following and click Register. You can put your own site URL if you don’t want to use it locally.
After registration
You’ll get an ID and Secrets like this, which you’ll need to use in your code, so make sure to save them in an environment variable file like .env
.
Now let’s utilize the libraries we installed earlier.
Create a file in the pages/api/auth/[...nextauth].js
path
import NextAuth from "next-auth";
import GithubProvider from "next-auth/providers/github";
export const authOptions = {
providers: [
GithubProvider({
clientId: process.env.CLIENT_ID,
clientSecret: process.env.CLIENT_PW,
}),
],
secret: process.env.JWT_SECRET,
};
export default NextAuth(authOptions);
Now let’s create a page that will use the login functionality.
The library takes care of creating the login page for us, so let’s create a button
component
Create the app/components/LoginBtn.jsx
file
"use client";
import { signIn } from "next-auth/react";
export default function LoginBtn() {
return (
<button
onClick={() => {
signIn();
}}
>
Log in
</button>
);
}
Create the app/components/LogoutBtn.jsx
file
"use client";
import { signOut } from "next-auth/react";
export default function LogoutBtn({ userName }) {
return (
<button
onClick={() => {
signOut();
}}
>
{userName} Log out
</button>
);
}
Let’s apply the two components.
app/layout.js
import "./globals.css";
import Link from "next/link";
import LoginBtn from "components/LoginBtn";
import LogoutBtn from "components/LogoutBtn";
import { authOptions } from "pages/api/auth/[...nextauth]";
import { getServerSession } from "next-auth";
export default async function RootLayout({ children }) {
let session = await getServerSession(authOptions);
let name = "";
if (session) {
name = session.user.name;
}
return (
<html lang="en">
<body>
<div className="navbar">
<Link href="/" className="logo">
Appleforum
</Link>
{session ? (
<Link href="/write">Write</Link>
) : (
<Link href="/register">Register</Link>
)}
<Link href="/list">List</Link>
{session ? (
<Link href="/">
<LogoutBtn userName={name} />
</Link>
) : (
<LoginBtn />
)}
</div>
{children}
</body>
</html>
);
}
Landing pages
After clicking the Log in button, you’ll see the following screen.