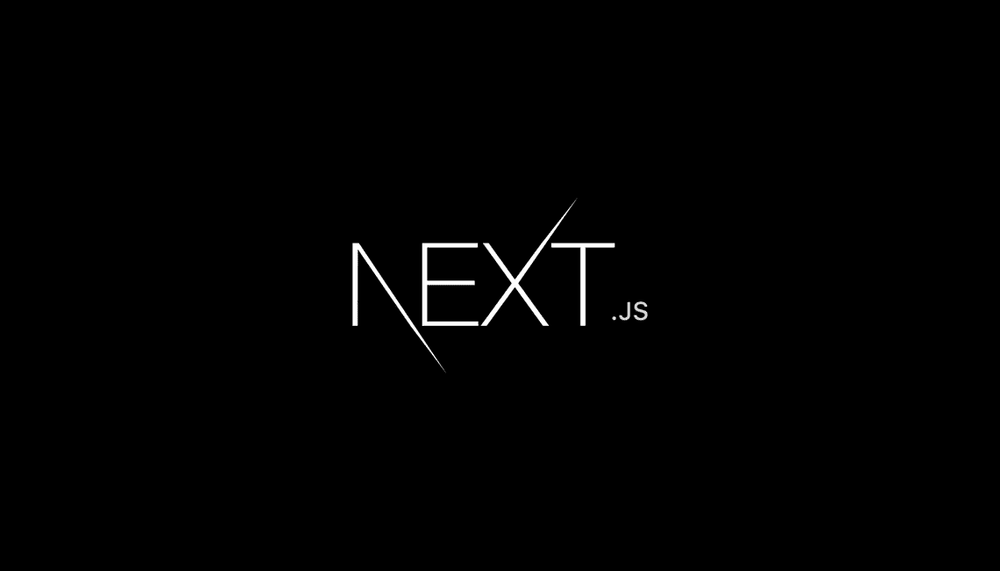
Social login with Auth.js 2
Next.js version
"next": "13.2.4”
Setting up the NextAuth library
npm install next-auth
I want to print the logged in user’s information on a page
For the client component
By importing
a <SessionProvider>
and wrapping it around the parent component, the child components have access to the useSession()
function.
(layout.js next to page.js)
'use client'
import { SessionProvider } from "next-auth/react"
export default function Layout({ children }){
return (
<SessionProvider>
{children}
</SessionProvider>
)
}
▲ If we create a layout.js
next to some page.js
and wrap it in <SessionProvider>
like this.
From now on, any client component
components that go inside of it will be able to print out the logged in user information.
(page.js)
'use client'
import { useSession } from 'next-auth/react'
export default function Page(){
let session = useSession();
if (session) {
console.log(session)
}
...
When you use useSession()
, it will leave the currently logged in user information in its place, so you can put it in a variable and use it.
However, it’s usually better to get the user information from the server component
and send it to the client component
.
This is because the useSession
function may run a beat after the html
has been displayed.
For the server component
import { authOptions } from "@/pages/api/auth/[...nextauth].js"
import { getServerSession } from "next-auth"
export default function Page(){
let session = await getServerSession(authOptions)
if (session) {
console.log(session)
}
If you get getServerSession()
and authOptions
and use them in your component, you’ll have the user information there.
You can put it in a variable like let session
and print it out.
Example using server component
export default async function RootLayout({ children }) {
let session = await getServerSession(authOptions);
let name = "";
if (session) {
name = session.user.name;
}
return (
<html lang="en">
<body>
<div className="navbar">
<Link href="/" className="logo">
Appleforum
</Link>
{session ? (
<Link href="/write">Write</Link>
) : (
<Link href="/register">Register</Link>
)}
<Link href="/list">List</Link>
{session ? (
<Link href="/">
<LogoutBtn userName={name} />
</Link>
) : (
<LoginBtn />
)}
</div>
{children}
</body>
</html>
);
}
The user’s name appears as text before Button
.
Social Login Disadvantages
However, since social login is a method that relies on a third-party site
- If that site becomes less popular over time, it can become obscure.
- If that site goes down, like the KakaoTalk server fire, social login is impossible.
But if you choose a reliable company like Google or Apple.
it doesn’t seem to matter if you create only one social login..