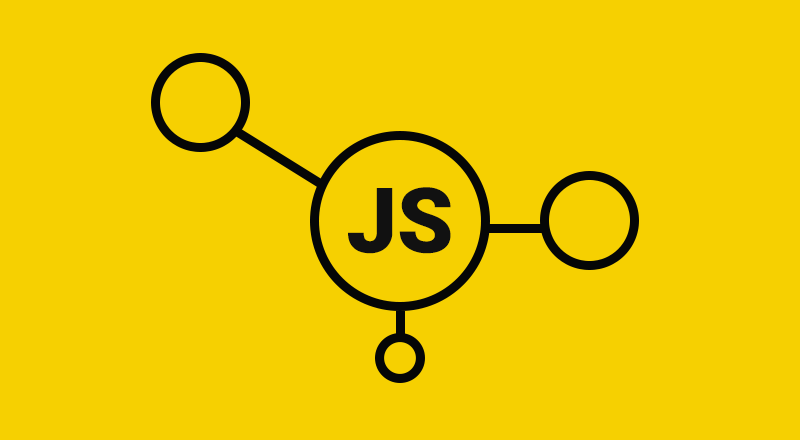
Let’s learn extends
, the ultimate JavaScript object-oriented syntax.
It’s not difficult, just a few more things to memorize.
Use extends
when you want to create a class
that inherits class
Let’s say you made a grandpa class
.
The grandfather class
has attributes named first and last name.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
}
Then new grandfather**()
Well, you can easily create a new object
by doing something like this, right?
However, this class
is so useful that I would like to create another class
similar to it.
Then, you can create another class
yourself and copy and paste the contents, right?
However, if there is too much to copy inside class
, the code will be too long.
So ancient developers created a grammar called extends
.
If you create a ‘class’ using this, you can copy and paste the contents of the existing ‘class’ as it is.
To put it simply, it is “a grammar that helps to inherit and create other classes
.”
So let’s create a father class
that inherits from the grandfather class
. Follow me.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
}
class father extends grandfather {}
extends
can be written like this:
Then, a class
called father
is created by copying and pasting the class
called grandfather as it is.
If you want to check whether a real class
has been created, you can test it like new father();
.
**new** father**('minso');**
If you do this, anobject
data with lastName and firstName will be created.
You have the same class
as your grandfather, right? End of extends
syntax!
But if you want to add a new attribute to class
called father
Of course, you can add content inside the father constructor
.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
}
class father extends grandfather {
constructor() {
this.age = 50;
}
}
This way, objects created when new father()
will have {lastName, firstName, age}
properties.
But this gives me an error.
I get an error that I need to use super
.
Then just write super
.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
}
class father extends grandfather {
constructor() {
super();
this.age = 50;
}
}
A strange function called super()
“means the constructor()
of the parent class
inheriting by extends
“. (memory items)
To put it simply, grandfather’s class
constructor()
is the same as this. (need to memorize)
So now you can add this
. or something like this without errors. (Memorization)
But you could pass a name
parameter to the constructor()
of your grandfather class
, right?
You have to specify it in the same way so that you can inherit all the properties of your grandfather exactly.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
}
class father extends grandfather {
constructor(name) {
super(name);
this.age = 50;
}
}
Just as the grandfather constructor()
had a name
parameter, the father constructor()
also followed it.
(Parameter naming is possible freely)
Now, if you enter a parameter when you do new father();
, it will enter the this.name
property.
So let’s predict.
Q. If you write var a = new father('minso');
at the bottom of the code above, what will the variable a
contain?
-
Did you guess
-
The variable named
a
is a newly created object from theclass
called the father. -
So, I inherited the
lastName, firstName
from my grandfather and theage
from my father. -
Then, put
'minso'
in place ofthis.firstName
and run it. So{ lastName : 'Kim', firstName : 'minso', age : 50 }
becomes an object.
-
If you add a method (function) to grandpa
If I add a function inside the grandfather class
, can the children of the father class
inherit it?
Could you try an experiment?
class grandfatehr {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
sayHi() {
console.log("Hello I'm grandpa");
}
}
class father extends grandfatehr {
constructor(name) {
super(name);
this.age = 50;
}
}
var a = new father("minso");
Then, can the object named a
use the function sayHi()
?
- You can write.
If an object named a
uses a.sayHi()
like this
-
Ask if the object named
a
hassayHi
-
If not, ask if
father.prototype
hassayHi
-
If not, ask if
sayHi
exists ingrandfather**.prototype
In this way, it looks up to run sayHi
.
But sayHi()
is a function added to grandfather.prototype
An object named a
can execute sayHi()
function.
But what if you want to inherit functions between classes
?
What do you mean… I want to create a function in a class
called father.
However, the sayHi()
function in the grandfather class
was very useful.
I want to bring this to my father ‘class’ as it is and use it.
What should I do?
You can also use super
here.
class grandfather {
constructor(name) {
this.lastName = "Kim";
this.firstName = name;
}
sayHi() {
console.log("Hello I'm grandpa");
}
}
class father extends grandfather {
constructor(name) {
super(name);
this.age = 50;
}
sayHi2() {
console.log("Hello I'm father");
super.sayHi();
}
}
var a = new father("minso");
If you use super
inside the prototype
function like that, it has a slightly different meaning from super
earlier.
super
here means prototype
of parent class
.
okay? super
has two meanings.
1. If used inside a constructor
, the constructor
of the parent class
2. If used inside a prototype
function, the prototype
of the parent class
no see. Oh, it’s hard to memorize, so let’s just know number 2 as a reference.
Q. So what will be printed to the console window when a.sayHi2()
is executed in the example code above?
-
Let’s predict exactly once and spread it out If you use
a.sayHi2()
, thesayHi2
function infather.prototype
will work.The function once executes
console.log("Hello I'm father")
andThe second line executes
super.sayHi()
. In other words, it is the same asgarandfather.prototype.sayHi()
It will runconsole.log("Hello I'm grandpa")
.So
"Hello I'm father"
,"Hello I'm grandpa"
are printed in the console window.