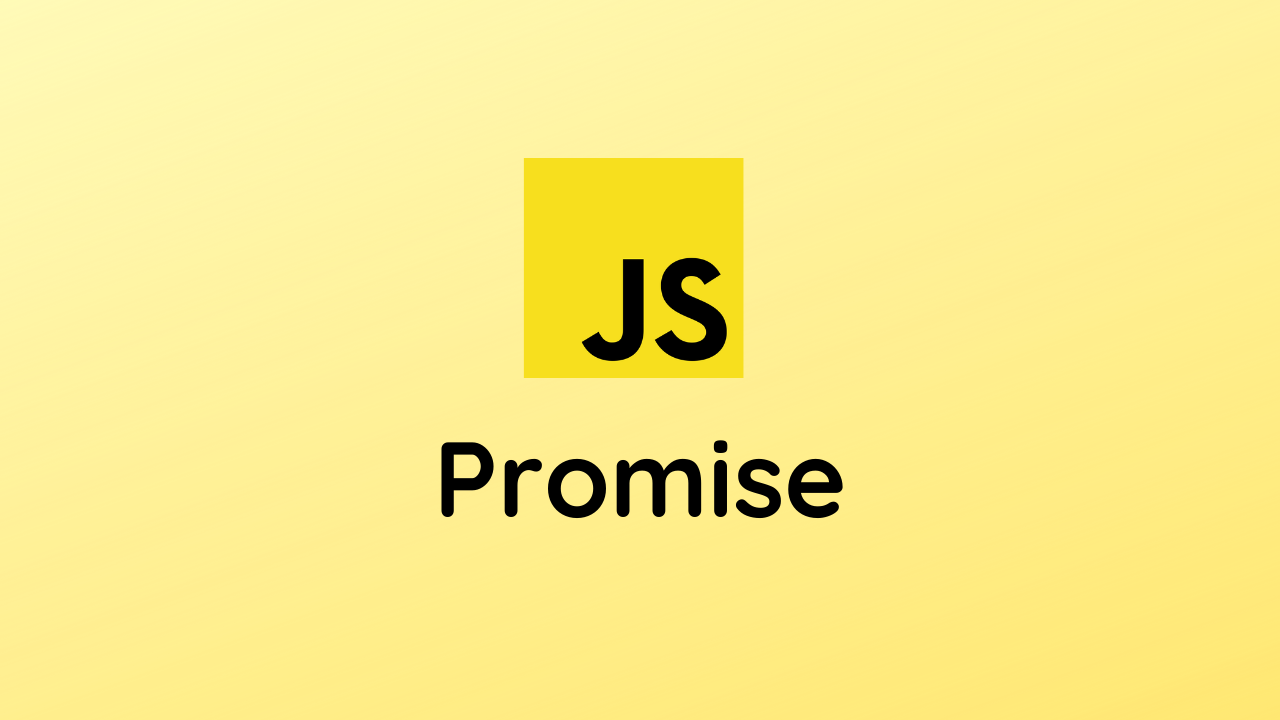
Those who do not like the callback function design pattern
You can use the Promise design pattern.
This isn’t a new feature of JavaScript, it’s just a code/function design pattern.
Try to use Promise
var pro = new Promise();
pro.then(function () {}).catch(function () {});
that’s it! If you create a variable object called Promise with the new Promise()
syntax, Promise production is finished.
Then, it can be executed by attaching then()
to a variable called a promise.
When the code inside the promise completes execution, the code inside the then()
function is executed.
If the code execution fails, the code in the catch()
function is executed.
Promises are a design pattern that helps you execute code sequentially in this way.
Promises are better than callback functions for two reasons.
-
Unlike callback functions, when executing something sequentially, the code does not grow sideways. Then function is attached to execute sequentially.
-
You can write code that says, ‘Please execute a specific code in case of failure’, which is impossible with a callback function. (catch)
Promise meaning and usage
Execute then() on success, catch() on failure~ Promise is what helps you write the code.
So, to easily define Promise, it is a success & failure judgment machine.
It’s easy to judge success/failure inside a promise.
var pro = new Promise(function (sucess, fail) {
sucess();
});
pro.then(function () {}).catch(function () {});
If you add a callback function in Promise()
, you can make a success/failure decision within it.
If you write the first parameter as success()
in the form of a function, it will be judged as success.
If you write the second parameter as fail()
in the form of a function, it will be judged as failure.
The above code is supposed to execute success()
unconditionally, so it judges success unconditionally.
After that, the code in then()
is executed.
Let’s see an example of actual use.
1. To run specific code after success?
var pro = new Promise(function (sucess, fail) {
var arithmetic = 1 + 1;
pro();
});
pro
.then(function () {
console.log("sucess");
})
.catch(function () {});
Added a function that performs mathematical operations in Promise()
.
And I added code to execute the success()
code when the operation is complete.
(General codes are executed one after the other if written side by side like this)
Inside the then function, you can put code to be executed when the promise succeeds.
Then the design is over.
-
Now, when the mathematical operation within the promise is completed, a
success()
decision is made, -
In case of success, the code within
then()
is executed.
In a promise, a failure decision can be made under certain circumstances.
var pro = new Promise(function (sucess, fail) {
var arithmetic = 1 + 1;
fail();
});
pro
.then(function () {
console.log("arithmetic sucess");
})
.catch(function () {
console.log("fail");
});
The moment the function called fail()
is executed, a failure decision is made.
Then the code in catch()
is executed.
In case of failure, it can execute other contents, so it is much more intuitive and useful than just a callback function design.
For reference, if you want to use the result of an operation in then
success()
Just put it inside a function.
var pro = new Promise(function (sucess, fail) {
var arithmetic = 1 + 1;
fail();
});
pro
.then(function (result) {
console.log("arithmetic sucess" + result);
})
.catch(function () {
console.log("fail");
});
2. wait 1 second to run specific code after success?
var pro = new Promise(function (sucess, fail) {
setTimeout(function () {
sucess();
}, 1000);
});
pro
.then(function () {
console.log("sucess");
})
.catch(function () {
console.log("fail");
});
A few features of Promises
-
You can see the current status by printing the variable created with new Promise() to the console window. Before the success/failure judgment,
<pending>
is displayed.After success
<resolved>
After failure,
<rejected>
is returned. -
Promises are not code that makes synchronous asynchronous. Promises have nothing to do with asynchronous execution. They’re just a kind of design pattern that can make coding pretty.