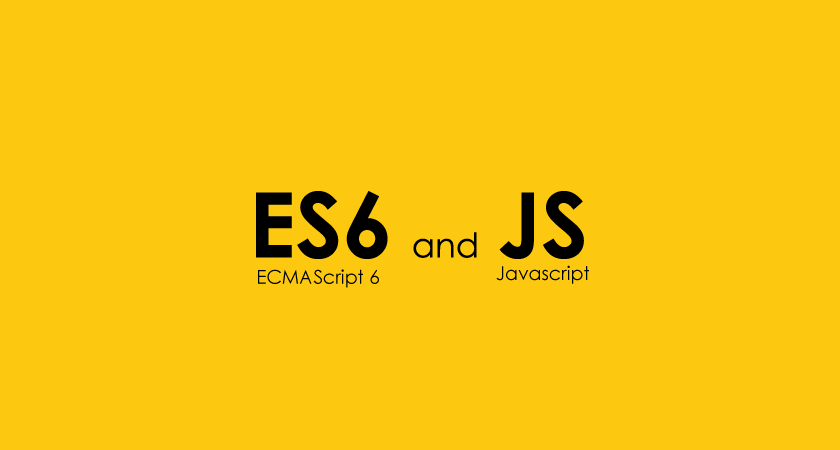
Let’s learn ES6 Spread Operator syntax
If you just take three periods in a row… that’s the grammar called the spread operator.
what role do you
“The operator that removes parentheses”.
Let’s attach it to the Array
.
var arr = ["hello", "world"];
console.log(arr);
console.log(...arr);
Then remove the parentheses attached to the array
called arr.
▲ If you attach the spread operator like line 3 and print it out, only ‘hello’ and ‘world’ with parentheses removed will be output to the console window.
When applied to a text, it removes parentheses attached to the text.
Where are the parentheses? In fact, characters are very similar to the array
datatype. You can see that there are invisible square brackets.
var str = "hello";
console.log(str[0]);
console.log(str[1]);
Did you know that str can be printed like an array
in this way?
Even the character hello
has the feeling of ['h', 'e', 'l', 'l', 'o']
surrounded by square brackets.
It’s not actually like that, it’s a feeling, a feeling
So what happens when we append spread
to a character?
Removes square brackets.
var str = "hello";
console.log(str);
console.log(...str);
If you print the third line of the code above, the characters h e l l o
are displayed in the console window.
console.log('h', 'e', 'l', 'l', 'o')
So, attaching spread
to a character spreads the alphabet one by one.
(or just memorize that it removes invisible square brackets
from text)
Where to use this is 1. It is used very often for merging/copying Arrays.
What if you want to combine two arrays?
var a = [1,2,3];
var b = [4,5];
var c = [How to make 1,2,3,4,5 come in here?];
It’s very easy with the spread operator
.
var a = [1, 2, 3];
var b = [4, 5];
var c = [...a, ...b];
After doing this, if you output an array
called c
, it becomes [1,2,3,4,5]
.
So let’s look at the case of array
copying.
▼ If you want to create b
by copying an array
called a
, wouldn’t you normally do this?
var a = [1, 2, 3];
var b = a;
console.log(a);
console.log(b);
The equal sign = makes it easy to put the value in a
into b
. ([1,2,3]
Copy complete!)
However, in JavaScript, if you copy like this, it will be a big deal.
If you copy with an equal sign, a
and b
variables do not have [1,2,3]
each, but value sharing occurs.
So, if you modify the array
of a
, a strange bug happens that b
is also changed in the same way.
var a = [1, 2, 3];
var b = [...a];
console.log(a);
console.log(b);
After using spread to remove the parentheses of the a
value, put the parentheses again.
If you do so, the value sharing of a
and b
variables will not occur as before.
As for where to use this, 2. It is used very often for merging/copying objects.
Guys, what if you want to combine two object
?
var o1 = { a : 1, b : 2 };
var o2 = { c : 3, and all of things of o1... }
I want to make o2
, but I want to take and add the contents of o1
as it is.
Then, don’t worry about how to do it, just think of spread operator
.
This operator removes braces as well as square brackets.
So if you write
var o1 = { a: 1, b: 2 };
var o2 = { c: 3, ...o1 };
console.log(o2);
If you output an object called o2
, it contains all the key values of a
, b
, and c
.
This is because the o1
object was added by removing the parentheses using the spread operator.
Note 1.
What happens when object key values are duplicated?
var o1 = { a: 1, b: 2 };
var o2 = { a: 3, ...o1 };
console.log(o2);
I want to create o2
by adding an item o1
, but the key value a
already exists.
If the value ‘a’ is duplicated like this, the a
that follows unconditionally wins.
So, if you print it out, it contains the data a : 1
.