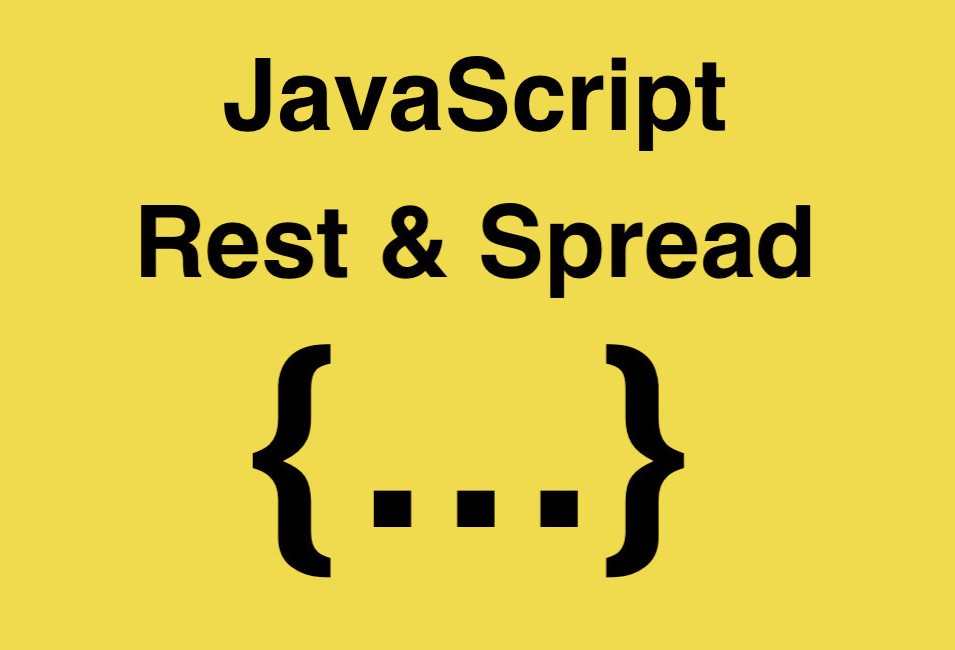
1. spread
problem
var a = [1, 2, 3];
var b = "ET";
var c = [...b, ...a];
console.log(c);
What is the output of the code above?
A
This will output an array
named ['E', 'T', 1, 2, 3 ]
.
If you `spread’ the letters, each letter is divided into commas.
spread
an array
removes square brackets.
2. spread
problem
var a = [1, 2, 3];
var b = ["you", "are"];
var c = function (a, b) {
console.log([[...a], ...[...b]][1]);
};
c(a, b);
What is the output of the code above with a lot of square brackets?
A
It tells us to put the data named a and b into the function c
and execute it.
The function of c
may seem complicated, but
If you look at these codes one by one, it’s not a big deal.
[ [...a], ...[...b] ][1]
where a and b are put
[ [1,2,3], ...['you', 'are'] ][1]
and removing spread
[ [1,2,3], 'you', 'are' ][1]
, and [1]
means the first data, so if you print it out
The text 'you'
appears in the console window.
3. default parameter problem
function fn(a = 5, b = a * 2) {
console.log(a + b);
return 10;
}
fn(3);
What is the output of the code above?
A
The function can take two parameters, and we are executing it by entering 3
.
It means that only one parameter was inserted in place of a.
Then, the parameter b will have the value a * 2
because the default parameter is triggered.
So a is 3 and b is 6.
‘9’ will be printed out.
4. default parameter problem
function fn(a = 5, b = a * 2) {
console.log(a + b);
}
fn(undefined, undefined);
What is the output of the code above?
A
If no parameter is input to the function, undefined
is displayed when the parameter is printed.
therefore
function fn(a) {
console.log(a);
}
fn();
The result of running this code is undefined
.
In JavaScript functions, the parameter automatically becomes `undefined’ if no parameter is passed.
So what happens if you deliberately enter undefined
as a parameter?
It is the same as not entering parameters.
So, the default parameter is triggered and outputs the result of 15
.
5. I want to create a function that creates an array
It’s impractical, but let’s try it.
I want to create a function that creates an array
as it is when data is entered as a parameter.
function arr(){
(What code can I put in here?)
}
var newArray = arr(1,2,3,4,5);
console.log(newArray);
Writing this should output [1,2,3,4,5]
.
If you put 100 numbers into the function, 100 numbers should be put in Array
.
How can I create a function called arr
? (Do not use new keyword)
A
Looks like you learned a good way to put all your parameters into an array
.
You can use rest
parameters.
function arr(...rest) {
return rest;
}
var newArray = arr(1, 2, 3, 4, 5);
console.log(newArray);
Writing this will output [1,2,3,4,5]
.
Also, no matter how many parameters you put in, an array
is created and output.
In the previous method, you can develop similarly by using a variable called arguments
.
6. Find the maximum value
If you want to find the maximum value in JavaScript.. you can use the built-in built-in function Math.max()
.
Math.max(5, 6, 4, 3);
If you write it like this, it will output the maximum value as ‘6’.
But there are quite a few numbers that I want to check the max value of.
var numbers = [2, 3, 4, 5, 6, 1, 3, 2, 5, 5, 4, 6, 7];
I want to put it in Math.max()
, but how should I do it?
A
If you want to put all data in array
in Math.max()
Wouldn’t it be better to unpack array
and put it in?
var numbers = [2, 3, 4, 5, 6, 1, 3, 2, 5, 5, 4, 6, 7];
console.log(Math.max(...numbers));
If you write like this, it will find the maximum value among array
data.
That’s it.
Why is it written in function parenthesis but not a rest parameter?
When declaring a function, ...
is a rest parameter
When using functions, ...
is the spread operator.
7. I want to create a function that sorts letters alphabetically.
First of all, when JavaScript wants to sort the data in array
alphabetically
It is used by appending the array
built-in function called sort()
. (applicable only to array
)
console.log(["b", "c", "a"].sort());
//['a', 'b', 'c']
You can easily sort by adding sort()
like this. That’s it.
However, we want to create an alphabetical sorting function that can be applied to strings as well as to array
.
function sortFn(){
(What code can I put in here?)
}
sortFn('bear');
If you use sortFn('bear')
What should I do if I want to make it output in alphabetical order like a b e r
in the console window?
(you can use the sort()
function)
A
You said you could use the sort()
function, but unfortunately sort
can only be used with array
.
Then, if you just make an array
of the characters, you can use the sort
function, so wouldn’t it be an easy solution?
Put the character bear
into an array like ['b', 'e', 'a', 'r']
and sort the alphabet.
then what should i do
function sortFn(str) {
console.log([...str].sort());
}
sortFn("bear");
With [...str]
, str
is unpacked with spread
and put back into array
.
Like ['b', 'e', 'a', 'r']
.
Then, sort this with sort()
and you’re done, right?
And if you want to put square brackets on the sorted data
function sortFn(str) {
console.log(...[...str].sort());
}
sortFn("bear");
Wouldn’t it be nice to write spread
there again?
Then, a b e r
will be printed in order in the console window. That’s it.
8. Create a data mining function
There is a function that data analysis people often create and use.
This function counts the number of occurrences of an alphabet. Let’s make one too.
Enter countStr**('aacbbb')**
in the console window.
{ a: 2, b: 3, c: 1 }
▲ I want to create a function called count() that prints like this.
To put it simply, it is a function that counts the number of alphabets in the input word, records it in an object, and outputs it.
How can I create a function called counting?
A
It’s no big deal, and you can run a loop over the text.
You can just use a loop, or you can use a forEach()
loop to make it simpler.
forEach()
is a function that can only be attached to array
.
If you want to attach it to a letter… wouldn’t it be possible to make the letter into an ‘array’?
function countStr(str) {
var result = {};
[...str].forEach(function (a) {});
}
So, if you insert letters into the function, use spread
to save each alphabet in an array.
And I ran a forEach
loop there.
Then, the repetition statement runs as many times as the number of each alphabet in the letter,
As the loop loops, the value a becomes each alphabet.
Now, since the loop runs as much as each alphabet, you can develop the function inside the loop.
If there is an item called result**[a]** (result.a)
in the object named result
, add 1 to the value
If not, please register as 1~ (a: 1 like this) I think you can write the code.
So I tried it.
function countStr(str) {
var result = {};
[...str].forEach(function (a) {
if (result[a] > 0) {
result[a]++;
} else {
result[a] = 1;
}
});
console.log(result);
}
(result[a]
is how to extract data from within an object.)
And I wrote a code to output the result to the console window after the loop runs.
It prints fine.