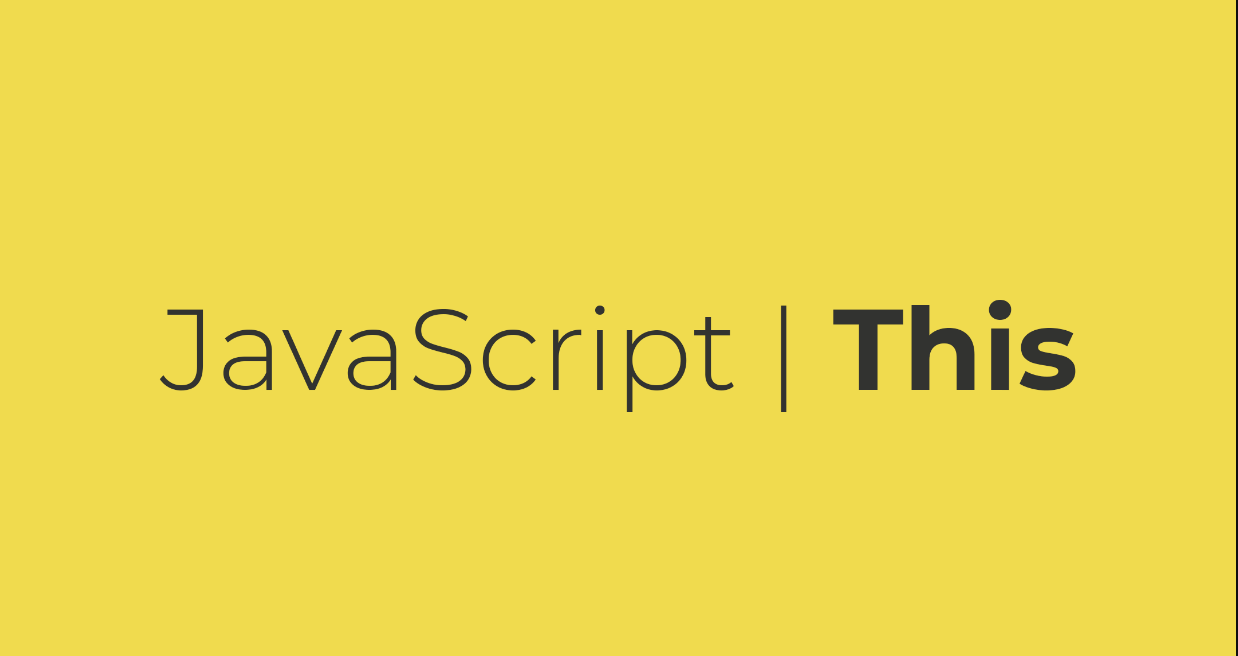
1-1. When used as is or inside a function, this refers to the window.
Just create any HTML file and open the <script>
tag in the middle
First of all, let’s print the keyword this to the console window.
console.log(this);
Then, the this keyword just returns window {something}
.
Similarly, if you call the value this
inside a normal function,
function fn() {
console.log(this);
}
fn();
Likewise, the value ‘this’ is output as ‘window’.
This is the first meaning of ‘this’. It is just window
.
Q. What is a window?
A. document.getElementById()
, alert()
, console.log()
A repository for these functions. An archive is nothing special, it’s just a big {object}
.
It also stores this value when you create a global variable.
<script>
var x = 300;
</script>
If you create a variable in a large space like this, a variable called x
is automatically created in a large object called window.
The same goes for functions.
1-2. When used inside a function in strict mode, this
is undefined
.
<script>
'use strict'; function fn(){console.log(this)}
fn();
</script>
In strict mode, declare a variable without the var keyword, or
Declaring variables with the strange keyword arguments or preventing such mistakes.
In strict mode, when the this keyword is called inside a general function, it is forcibly set to the value of undefined.
2. There can be functions within the object data type, where the this
value means ‘master’.
var obj1 = {
data: "Kim",
fn: function () {
console.log("Goood");
},
};
Just like inserting letters or numbers, you can insert a function like that into an object.
So, how do you use the function
var obj1 = {
data: "Kim",
fn: function () {
console.log("Goood");
},
};
obj1.fn();
You can write it like this. Then, the word 'Goood'
will be printed in the console window.
Functions that enter an object are called methods in the jargon that seems to exist.
However, if you use this inside a method, you get a mysterious value. It’s the price of being the owner
var obj1 = {
data: "Kim",
fn: function () {
console.log(this);
},
};
obj1.fn();
What would happen if the keyword this was printed instead of the word Goood
?
{ data: 'Kim', fn function: f }
Why isn’t this value displayed in the console window?
What this is is just obj1
that you just created.
So, if you use this in a method, this means the object that has the method.
If you want to memorize it easily, this refers to ‘the owner of the method’.
When you create a function or variable roughly inside a script tag, the function or variable is not just created.
<script>function fn(){console.log()}</script>
The fn()
you just created is automatically added to an object called window to manage global variables or global functions.
So both code (1) and code (2) are the same story from the JavaScript point of view.
<script>
(1) function fn(){console.log(this)}
(2) window.fn = function(){console.log()};
</script>
(2) The syntax is just a syntax for adding function data to an object
called window. There is nothing difficult.
Anyway, the bottom line is that if you create a global function or a global variable, it will be put inside the window {object}
like that.
Even if we don’t do it on purpose, if we make it into a variable or function, JavaScript automatically puts it in the window.