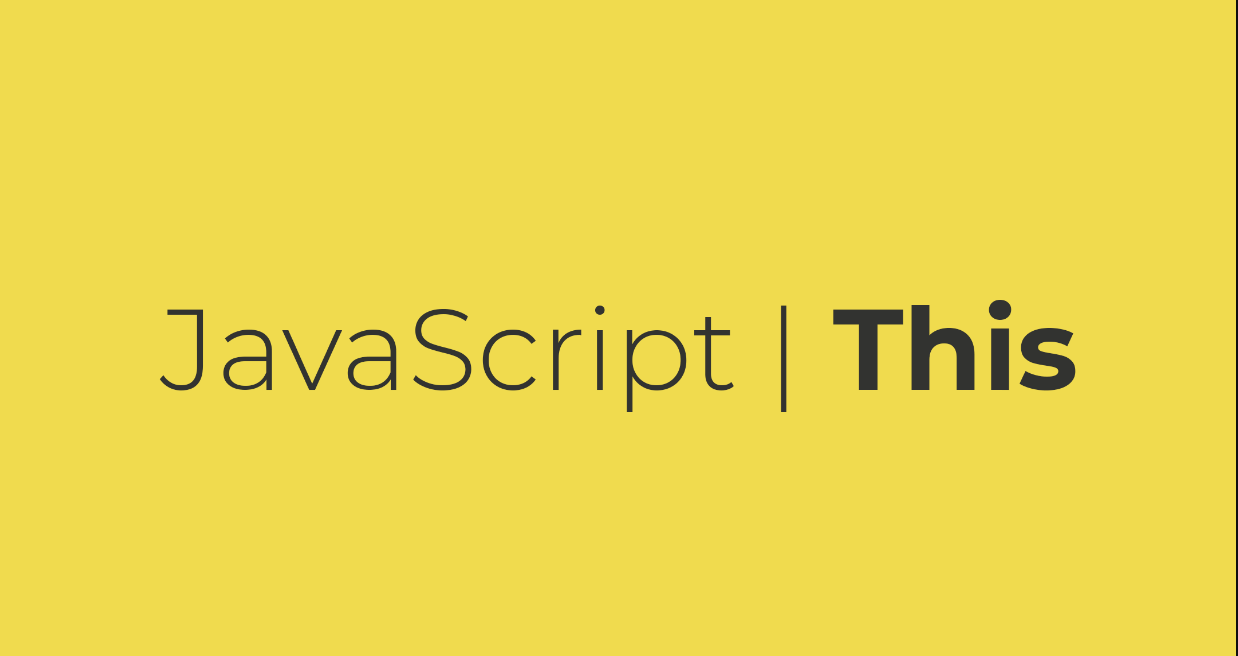
To clarify the meaning of this
-
If you just use it in a normal function, window
-
An object that operates a function when used in a function (method) within an object
3. When used inside a constructor
, it means a new object
created by the constructor
.
If you want to create multiple similar objects in JavaScript
Instead of copying the object, create a constructor
and use it.
To put it simply, a constructor is a machine that copies and creates objects.
Let’s see how to build a machine.
function machine() {
this.name = "Kim";
}
Here’s how to make a machine.
After making it using function syntax, inside this.Just add something
Here, this refers to objects that will be newly created from the machine.
So what does this.name='Kim'
mean?
It means “Please insert the value 'Kim'
into the name key value of the newly created object.”
▼ This is how to make an object in a machine that is good to know for reference.
function machine() {
this.name = "Kim";
}
var obj = new machine();
You can use the new
keyword to retrieve a new object
.
4. When used inside an eventlistener, this means e.currentTarget.
document.getElementById("button").addEventListener("click", function (e) {
console.log(this);
});
If you call this here, it has the same meaning as e.currentTarget
.
e.currentTarget is where the current event is triggered
Very simply, you mean the HTML element that is now attached with addEventListener
.
If in doubt e.currentTarget, this, document.getElementById(‘button’) You can print these three separately.
This is the final meaning of this.
case 1. If you use a callback function inside an event listener, what is this?
document.getElementById("button").addEventListener("click", function (e) {
var arr = [1, 2, 3];
arr.forEach(function () {
console.log(this);
});
});
I used a loop called forEach()
inside the event listener.
When using forEach()
iteration statement, function(){}
callback function must be inserted and used. so i put it in.
Q. What will the output of this
in the code above say?
According to the 4th meaning.. It was not written inside the eventlistener.
The inside of the eventlistener is correct, but the meaning changes because we encounter one more function inside it.
According to the 3rd meaning.. I don’t think it’s a constructor.
Actually, the first or second meaning of this
is correct.
The callback function that exists like that is just the same as a normal function, so a window is displayed.
case 2. If a callback function is used inside an object, what is this?
var obj = {
names : ['kim', 'lee', 'park'];
fn : function(){
obj.names.forEach(function(){
console.log(this)
});
}
}
Names and data called functions are stored in objects called obj, respectively.
I ran a forEach loop in the data called a function,
Q.Then, what will come out if you print the value of this in here?
Since the function inside forEach()
is a normal function, a window
appears.
So this value can change each time you encounter a function.
Sometimes it’s hard to write what you want.
In that case, please change the function to an arrow function
.
var obj = {
names : ['kim', 'lee', 'park'];
fn : function(){
obj.names.forEach(() => {
console.log(this)
});
}
}
There is an arrow function syntax called () => {}
that can be used instead of function () {}
.
This is the same syntax to create a function.
This is useful when using this because it does not change the value of this inside the function.